Before and After image script
Description: Nothing ever stays the same, which is why we created this Before and After script to easily view an "after" version of any image as an overlay of the original one. It makes for a dramatic way of comparing two images and any subtle differences. A draggable handle bar lets the user decide how much of each image he/she wishes to view. The script supports the following two nifty features:
-
The "before" and "after" slides can each contain not just a main image, but other HTML as well, such as a caption.
-
The script can load another set of images on demand.
Use the Before and After image script on any set of images where you wish to highlight the difference between the old and new shot, such as pictures of a model pre and post touch up, an picture after witty annotates had been inserted, or a subject after striking weight lost. The possibilities are countless.
Demo:
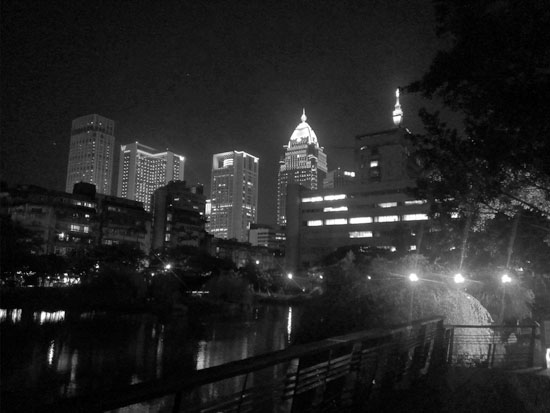
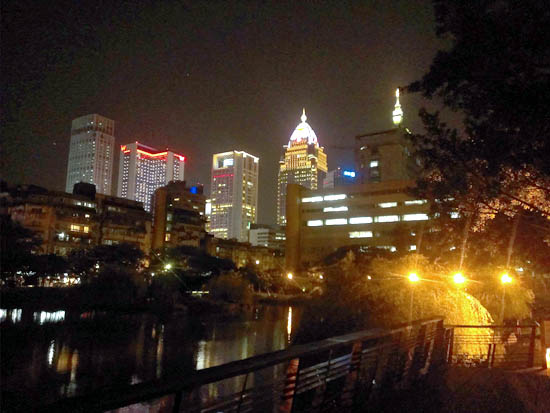
Step 1: Add the below code inside the <HEAD> section of the page:
The above code references two external files which you should download below (right click, and select "Save As"):
Step 2: Insert the below sample code into the BODY section of your page:
The code of Step 2 illustrates the HTML markup of a before and after image set up, which looks like the following:
<div id="baf" class="beforeandafter">
<div class="before">
<img src="before.jpg" />
<span class="caption">BEFORE</span>
</div>
<div class="after">
<img src="after.jpg" />
<span class="caption">AFTER</span>
</div>
</div>
It should consist of an outer container carrying an unique ID
and CSS class of "beforeandafter
". Inside this DIV should be two
child DIVs with class names "before
" and "after
". It
is inside each of these DIVs that you define the HTML to be shown for the "before
"
and "after
" frames. Each frame should consist of a single <IMG> tag along
with additional arbitrary bits of HTML such as a caption DIV, SPAN etc to show.
Initialization of the script
To initialize the script to display an instance of Before and
After image script, call new
ddeforeandafter()
in the HEAD section of your page after the document has
loaded:
jQuery(function(){ // after DOM has loaded
uniquevariable = new ddbeforeandafter({
//comma separated options here
})
})
Where "uniquevariable
" should be an arbitrary but unique variable
(for each instance of Before/After image script), and options is an object literal
containing the desired settings. For example:
jQuery(function(){ // after DOM has loaded
uniquevariable = new ddbeforeandafter({
wrapperid: 'baf',
revealduration: 1.5
})
})
Here's an explanation of each option:
options | Description |
wrapperid: string Required |
The ID of the main DIV container for this instance of Before and After Image script. |
initialreveal: ['amount', duration] Defaults to ['30%', 1] |
Sets the amount of the "after" image to
reveal automatically and the duration it takes when the page loads. The
first parameter 'amount ' should be a percentage string
between '0' and '100%', while the duration parameter is an
integer indicating the duration in seconds. The following setting
for example would have the "After" image cover 80% of the original image
by default after an animation of 2 seconds:
|
revealduration: duration Defaults to 0.4 |
The duration of the reveal effect each time the container is clicked on to reveal the desired portion of the "after" image, in seconds. Defaults to 0.4 (seconds). |
hidedragbardelay: duration Defaults to 1 |
Sets the delay after the mouse moves out of the "before/after" image container before the draggable bar is faded out to obscure it from view, in seconds. |
dragUI: 'string' Defaults to '<div class="drag"><div class="draghandle"></div></div>' |
Sets the HTML for the draggable bar
interface, which in general you should not need to edit. The modify its
style, edit ddbeforeandafter.css,
specifically, the "drag " and "draghandle "
classes for color, dimensions etc.If you wish to edit the interface's
HTML, maintain the default CSS classes used and the markup hierarchy,
specifically, the |
initialrevealeasing: 'string' Defaults to 1'cubic-bezier(0.390, 0.575, 0.510, 1.650)' |
Sets the CSS3
transition-timing-function value used to animate the "after"
image into view when the page loads (also see the
initialreveal
option above). The acceptable value is
any string valid to this CSS3 property. The default value specifies
a bezier function that creates a push pull effect.
See this page
to generate a different custom bezier based effect. |
Once the script is initialized, it also exposes two methods to update it on demand. The methods are:
method | Description |
scriptinstance.unveil(amt, [dur]) |
Reveals the "after" image by a certain amount
on demand. scriptinstance should be the unique but
arbitrary variable name you set new ddbeforeandafter() to
when initializing the script. The unveil() function
supports 2 parameters:
The below creates two links that set a "before/after" image to fully hide and reveal the "after" image, respectively: <a href="#" onClick="uniquevar.unveil('0%');
return false">Hide after image</a> |
scriptinstance.reload(beforeafterhtml,
dimensions) |
Reloads the contents of a Before
and After image script instance or change its dimensions on demand.
scriptinstance should be the unique but arbitrary variable name
you set new ddbeforeandafter() to when initializing the
script. The reload() function supports the following two parameters:
Either options can be set to // update before and after images // update container dimensions only // update both images and dimensions
|
Reloading a before/after image script instance with a different set of images
When calling
scriptinstance.reload()
to update the set of images shown
by a "Before/After" script instance, it's easier to define an outer function
that in turn calls the reload()
method with the desired parameters, for example:
<script>
function updatebeforeafter(){
bafinstance.reload(['<img src="newbefore.jpg" /><span
class="caption">New Before</span>', '<img src="newafter.jpg"
/><span class="caption">New After</span>'])
}
</script>
We'll use the above function with the following "Before/After" image instance to update its images when a link is clicked on:
<script>
jQuery(function(){ // after DOM has loaded
bafinstance = new ddbeforeandafter({
wrapperid: 'baf'
})
})
</script>
<div id="baf" class="beforeandafter">
<div class="before">
<img src="before.jpg" />
<span class="caption">BEFORE</span>
</div>
<div class="after">
<img src="after.jpg" />
<span class="caption">AFTER</span>
</div>
</div>
<a href="#" onClick="updatebeforeafter(); return false">Update
before and after images</a>